Service Workers
A step towards progressive web apps
The service worker is the core technology used in Progressive Web Applications. Without service workers, a website is just a website, just add a service worker, you now have an application.
What is a Service Worker?
A service worker is one type of web worker. It’s just a script file that runs separately from the main browser thread, enabling features like network requests, caching or retrieving resources from the cache, and push notifications, etc.
Service workers are fully asynchronous. They can’t access the DOM but can intercept all network requests. They can receive push messages from a server when the app is not active. Also, service workers can only work on secure connections, which means HTTPS. This is because service workers are very powerful and they can do serious damage to our system or network if they are tampered with by man-in-middle attack. But in development, we can use a local server.
How does service workers work?
A service worker sits between the browser and the network, acting as a proxy server. Service workers are event-driven and always live outside the browser process. The service worker is a script that executes in a thread making a website to perform better. It gets terminated when not in use and gets restored whenever required.
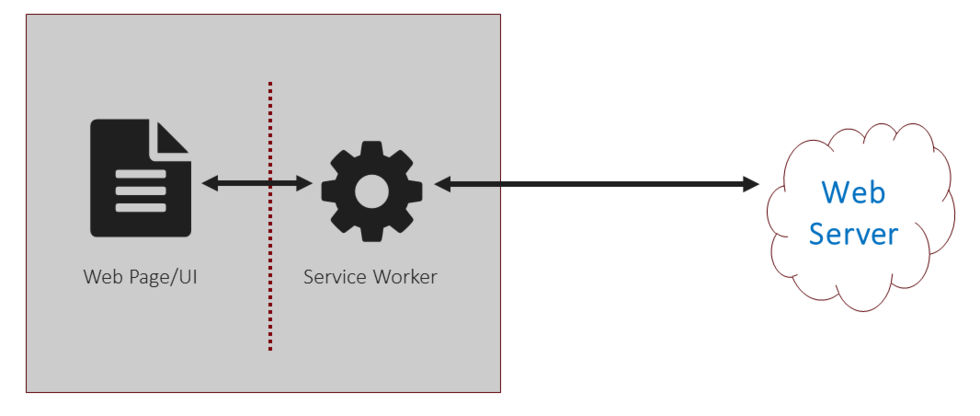
The first power a service worker brings to a website is the ability to enable offline capabilities. This is done with caching API. Caching not only enables offline experiences to a website but the caching resources will make content load faster under most of the network conditions.
To make application work offline, the service worker depends on two APIs:
- Fetch (for retrieving content from the network)
- Cache (persistent storage for application data).
Service Worker lifecycle
A service worker lifecycle includes:
- Registration
- Installation
- Activation
1. Registration and scope:
To install a service worker, we need to first register it in our main JavaScript code. This service worker registration tells the browser where the service worker is located, and to start installing it in the background.
Let’s look at an example:
// app.js file if('serviceWorker' in navigator) { navigator.serviceWorker.register('/service_worker.js') .then((registration) => console.log('Registration scope:', registration.scope); ) .catch((error) => console.log('Registration failed:', error); ); }
Here, we are first checking whether our browser supports the service worker or not. Then we are registering our service worker by the register() method in which we’ll pass our service worker file, which returns a promise that resolves when the service worker has been successfully registered.
In service workers, the scope is very important. The scope of the service worker determines the files that it can control. In simple words, it tells from which path the service worker will intercept requests. The location of the service worker file is the default scope.
2. Installation:
Once the registration of the service worker is done, the installation process starts. A service worker installation happens for the very first time when the service worker is new to the browser, as the browser or site doesn’t have a service worker registered previously. A service worker installation triggers an install event and we can perform our tasks when the service worker installs.
We can install a service like this:
// service_worker.js self.addEventListener('install', (event) => { console.log('Service Worker installed successfully!'); // perform some task });
3. Activation:
Once a service worker has successfully installed, next comes the activation stage. If the previous service worker is still running, then the new service worker enters a waiting stage and activates only when there are no pages that are using a previous service worker. This helps in ensuring that only one version of the service worker is running at a given time.
An activate event gets triggered when the new service workers activate. Here we can add code to clean up caches.
// service_worker.js self.addEventListener('activate', (event) => { console.log('Activating service worker...'); // perform some task });
Once activated, the service worker controls all pages that load within its scope and start listening for events from those pages. However, pages in our app that were loaded before the service worker activation will not be under service worker control.
As I specified above, service workers are event-driven, both the installation and activation processes trigger the corresponding install and activate events to which the service workers can respond.
To examine service workers, open Developer Tools, and navigate to the Service Worker section in the Application tab.
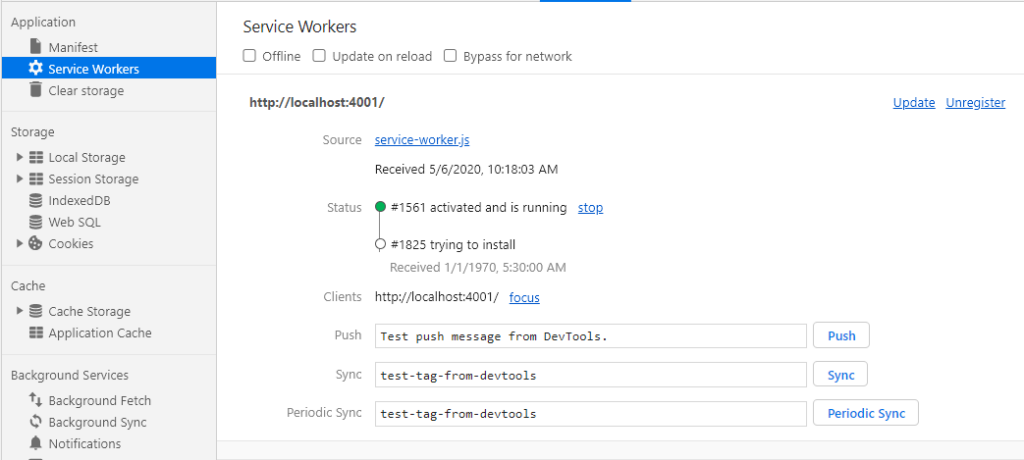
Service workers can speed up the repeated visits to our web app but make sure that our users have the best first-visit experience. We can ensure that by delaying the service worker registration until after the page has loaded during the first visit. We’ll still get all the benefits of having a service worker for our repeat visits.
A straightforward way to delay our service workers registration can be done as:
// app.js file if('serviceWorker' in navigator) { window.addEventListener('load',() => { navigator.serviceWorker.register('/service_worker.js') .then((registration) => console.log('Registration scope:', registration.scope); ) .catch((error) => console.log('Registration failed:', error); ); } }
I hope this article helped you in understanding the service workers. Now you can start building your PWA with the help of a Service worker.
Enjoy Coding
Akshata Waghe
- 10 backend mistakes (and their fixes) you must avoid as a Backend Developer
- 5 Frontend Trends You Can’t Ignore in 2022