Async React Hooks
In react we deal with the different types of components. It could be a class component or functional component. If it is a class component then we know how to use the lifecycle method in react, but if it is a functional component then we don’t have access to lifecycle methods. We have to use the useEffect() hook provided by React. But how to use async and await inside useEffect() hook is the challenge. So in this blog, I am going to cover how to use async react hooks.
Let’s start with an application that will fetch data from an API. The API will give data which will have the URL for GIF. User has to search the GIF according to name for that user have to enter a value in the search bar. We will get that data and show all the GIF’s related to our search value.
Note: You can get the GIF’s API from https://developers.giphy.com
Create a new React application
$ npx create-react-app async-react-hook $ cd async-react-hook
Now, we are ready with boilerplate code for our react application. Now we will start writing code for our application. To use hooks we will use the functional component of react.
Replace the code which is present in App.js with the following code.
import React from "react" function App() { <div style={{ display: "flex", flexDirection: "column", height: "100vh", alignItems: "center", justifyContent: "center"}}> <input style={{ width: "300px", height: "50px", borderRadius: 10, border: "1px solid grey", outline: "none", marginBottom: 20, padding: "0px 25px" }} type="text"/> </div> export default App;
In the above code, we have created a functional component with the name App. We have One search bar. The user has to enter some value or name in the search bar when the user enter something he/she will get all the related GIFs. If the user enters something wrong or not valid value it will show error GIF’s not found.
Now, we will create a hook to save our search value. Add the following line in our App.js file inside App function. To create a new hook we have to import useState hook from react.
import React, { useState } from "react"; function App(){ const [name, setName] = useState(""); ... }
Now, we have a hook that will store the value of our input or change the value. We will add the onChange function in our input tag. When we type something it will change the value of input or name accordingly.
Just change the code for the input search bar in App.js
... return ( ... <input style={{ width: "300px", height: "50px", borderRadius: 10, border: "1px solid grey", outline: "none", marginBottom: 20, padding: "0px 25px" }} type="text" value={name} onChange={(e) => setName(e.target.value)}/> ... )
We have our search bar ready. Now, we will fetch the data or GIF from the API.
Now, we will use useEffect hook to fetch the GIFs related to our search. useEffect hook is like lifecycles methods of react. We can use multiple useEffect hooks. It will load before the return function of our component. So we will write a code to fetch all GIF’s in useEffect and save to one state. We will create another hook name as “gif”, its initial value is an empty array.
Add following code in App.js file
function App(){ const [gif, setGif] = useState([]); ... }
We will add code to fetch data from GIPHY API. To call an API and get the data from the different servers this operation will take some time. To perform this operation we have to use async and await. We have to fetch the GIF’s when our component is loaded. If we don’t have any value to search then it will show the message “result not found”. When we enter something and value matches with the valid GIF’s name then we will get the result of all the GIFs related to that search.
To perform fetch all the GIF’s we will write code inside useEffect hook. As I said useEffect hook works like a lifecycle method of react. So we will write a code to fetch all GIF’s inside of useEffect hook.
Add the following lines in App.js file
function App(){ ... useEffect(() => { console.log("Inside useEffect"); }); ... }
We have added useEffect hook. Now run the application. To run the application using the following command.
$ npm start
When you start your application open the console in your browsers developer tools and you will find the line which we have console inside useEffect hook. useEffect hook takes exactly two arguments, second is optional. The first argument is callback function or the second argument is the dependency array.
We just check whether our useEffect function is working or not. Now we will add the code to fetch the GIF. We have to use async and await inside useEffect hook. If you use useEffect hook like the following then you will get some error.
useEffect(async () => { …some code here })
Now, we will see how to use async and await inside useEffect hook. Replace the following block of useEffect hook with the previous one.
useEffect(() => { async function getGif(){ const data = await fetch(`https://api.giphy.com/v1/gifs/search?api_key=7rXEjduHI4sQRH5jFi3hqLqtguk3o4k2&q=${name}&limit=25&offset=0&rating=G&lang=en`); const allGifs = await data.json(); setGif(gif.data) } getGif(); })
The above code in useEffect will fetch the GIFs according to search and set to gif state. Now we will use gif state to show all the fetch GIF. To render all the GIF’s add the following code in return after the input search bar.
function App(){ ... return( ... <div style={{ display: "flex", flexWrap: "wrap", height: "auto", backgroundColor: "blue"}}> { gif.length === 0 ? <p>Content not Found related to search...</p> : gif.map(g => <video autoplay style={{ width: 200, height: 200 }} controls key={g.id}> <source src={ g.images.preview.mp4 } type="video/mp4"/> </video> )} </div> )}
But there is one problem in the above code. As I said useEffect function takes exactly two arguments first is callback and second is dependency. Now in our application, we didn’t pass any dependency, so our useEffect function will execute each and every time. But we want to execute our useEffect function only when our name value will change. So we will pass the name as a dependency in useEffect.
Replace the useEffect hook code with the following code.
useEffect(() => { async function getGif(){ const data = await fetch(`https://api.giphy.com/v1/gifs/search?api_key=7rXEjduHI4sQRH5jFi3hqLqtguk3o4k2&q=${name}&limit=25&offset=0&rating=G&lang=en`); const allGifs = await data.json(); setGif(gif.data) } getGif(); }, [name])
Now, run the application and type something in the input box. You will get the following output.
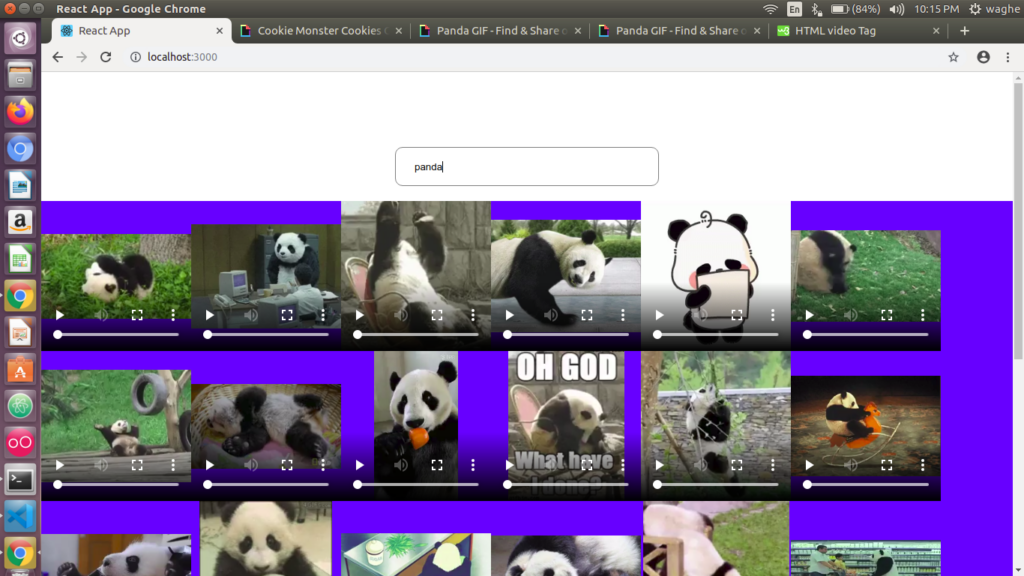
Final code of in App.js file
import React, { useState, useEffect } from "react"; function App() { const [name, setName] = useState(""); const [gif, setGif] = useState([]); useEffect(() => { async function getGif(){ const data = await fetch(`https://api.giphy.com/v1/gifs/search?api_key=7rXEjduHI4sQRH5jFi3hqLqtguk3o4k2&q=${name}&limit=25&offset=0&rating=G&lang=en`); const allgif = await data.json(); console.log(allgif.data); setGif(allgif.data); } getGif(); }, [name]); return ( <div style={{ display: "flex", flexDirection: "column", height: "auto", alignItems: "center", justifyContent: "center", paddingTop: 100 }}> <input style={{ width: "300px", height: "50px", borderRadius: 10, border: "1px solid grey", outline: "none", marginBottom: 20, padding: "0px 25px" }} type="text" value={name} onChange={(e) => setName(e.target.value)}/> <div style={{ display: "flex", flexWrap: "wrap", height: "auto", backgroundColor: "blue"}}> { gif.length === 0 ? <p>Content not Found related to search...</p> : gif.map(g => <video style={{ width: 200, height: 200 }} controls key={g.id}> <source src={ g.images.preview.mp4 } type="video/mp4"/> </video> ) } </div> </div> ); } export default App;
Conclusion:
We have learned how to use async and await inside useEffect hook.
Team Udgama
- 10 backend mistakes (and their fixes) you must avoid as a Backend Developer
- 5 Frontend Trends You Can’t Ignore in 2022