Introduction to Next.js
Framework for building server-rendered React applications
Next.js is a new framework for server-rendered React applications.
Next.js is a react framework for building:
- Static websites
- Mobile web apps
- Desktop applications
- Enterprise Applications
- Lightweight Applications
Why should we care about Next.js?
- It is easy to get started building a project with Next.js after installing all the required packages.
- It allows code-splitting. As a programmer, we know how important code splitting is to ensure an application’s performance is optimal. As the name suggests, code splitting allows us to split our code to create a series of lightweight bundles.
- It has built-in Typescript support.
- Next.js allows all of our optimized bundles of code to be lazy-loaded behind the scenes.
- Instead of having to configure webpack and babel, we can rely on Next.js.
- Easy to build server-rendered react applications.
- It provides a page-based routing system.
- It has built-in CSS and Sass support, and support for any CSS-in-JS library
- It provides hot code reloading. Next.js reloads the page whenever it detects any change.
How to get started?
We have two ways of building the Next.js application.
- One way of creating Next.js application is by using create-next-app:
create-next-app is a tool for setting up the Next.js application in one simple command as
npx create-next-app my-app
This will produce a boilerplate code in a folder named my-app. The boilerplate code will have pages and static folders. Each file inside the pages folder corresponds to one specific route of your application and a static folder is for serving static files.
- Another way of creating Next.js application is by building a project manually by yourself. For creating it manually, we have to first create a project directory. We’ll name it as nextjs-app.
mkdir nextjs-app
Then we’ll create a package.json file in the root directory of our project.
cd nextjs-app npm init -y
After our package.json file is created, we’ll install the required dependencies inside our project directory.
npm install next react react-dom //or yarn add next react react-dom
Now, create two folders as pages for serving routes and static for serving static files.
mkdir pages static
Inside the pages directory, we will create an index.js file for our main route.
// pages/index.js import React from 'react'; class App extends React.Component { render() { return( <div> <h2>Welcome to my first Next.js Application!!</h2> </div> ) } } export default App;
To run our application, we need to add a script in our package.json file as
// package.json "scripts": { "start": "next -p 5000" }
Now, run the application using
yarn start npm start
Our application is running on port 5000 and it will look like:
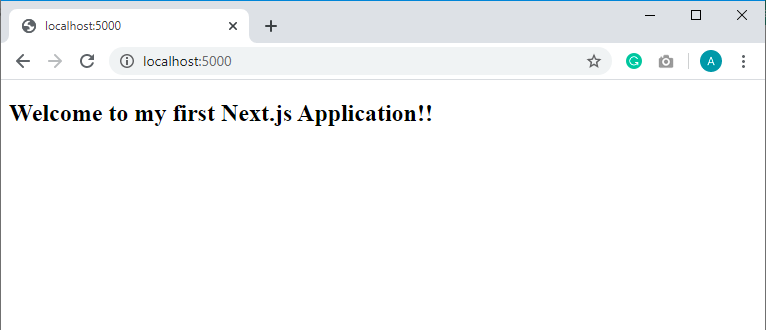
You can choose any other port or default port 3000 to run your application.
Now we will add one more page for navigating to about route.
// pages/about.js import React, { Component } from 'react'; import Link from 'next/link'; class About extends Component { render() { return( <div> <h1>Welcome to About page</h1> <Link href="/">Back to the Main page</Link> </div> ) } } export default About;
Now, we have our about page ready where we have added a link for navigating to the main page. So we’ll update our index.js file to navigate to about page.
// pages/index.js import React from 'react'; import Link from 'next/link'; class App extends React.Component { render() { return( <div> <h2>Welcome to my first Next.js Application!!</h2> <Link href="/about">Go to the About page</Link> </div> ) } } export default App;
Now again run the application, and you’ll have the links navigating to both the pages.
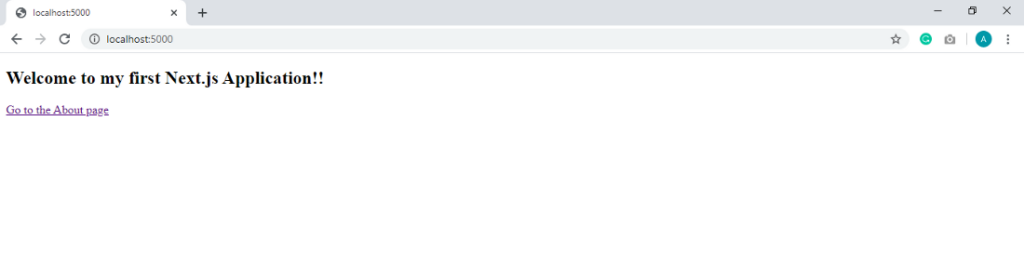
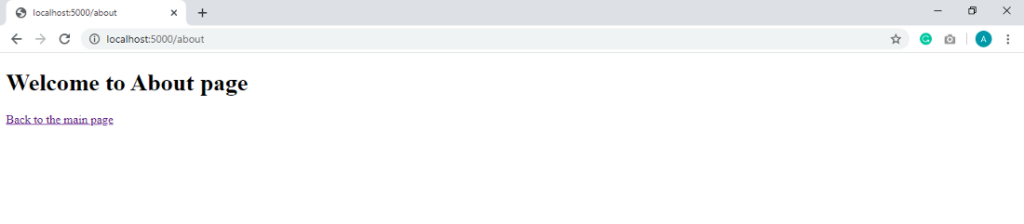
Yeah, We have successfully created a simple Next.js application. Now it’s your turn to create your amazing react application with Next.js for a better experience.
Enjoy Coding