Using ESLint and Prettier to work with a Typescript Project
Linting is the automated process of checking whether there are any bugs in your code or not. In short, it does the work of the human eye but just with more efficiency. It’s done by a lint tool also called linter.
Prettier is another automated process for formatting your code to follow consistent styling. It makes your code look good as said prettier.
When it involves linting TypeScript code, there are two linting tools: TSLint and ESLint. TSLint supports the sole TypeScript, whereas ESLint supports both JavaScript and TypeScript.
In the Typescript 2019 roadmap, the TypeScript core team explains that ESLint incorporates a more performant architecture than TSLint which will only be focusing on ESLint when providing editor linting integration for TypeScript. So it’s recommended to use ESLint for a typescript project.
Let’s first install ESLint and Prettier :
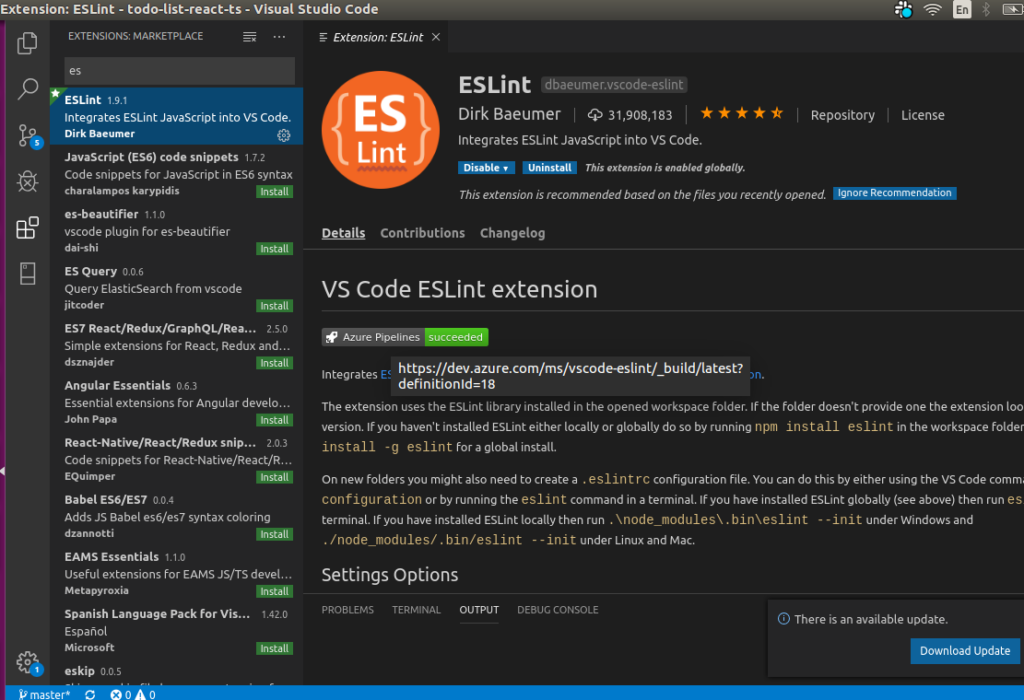
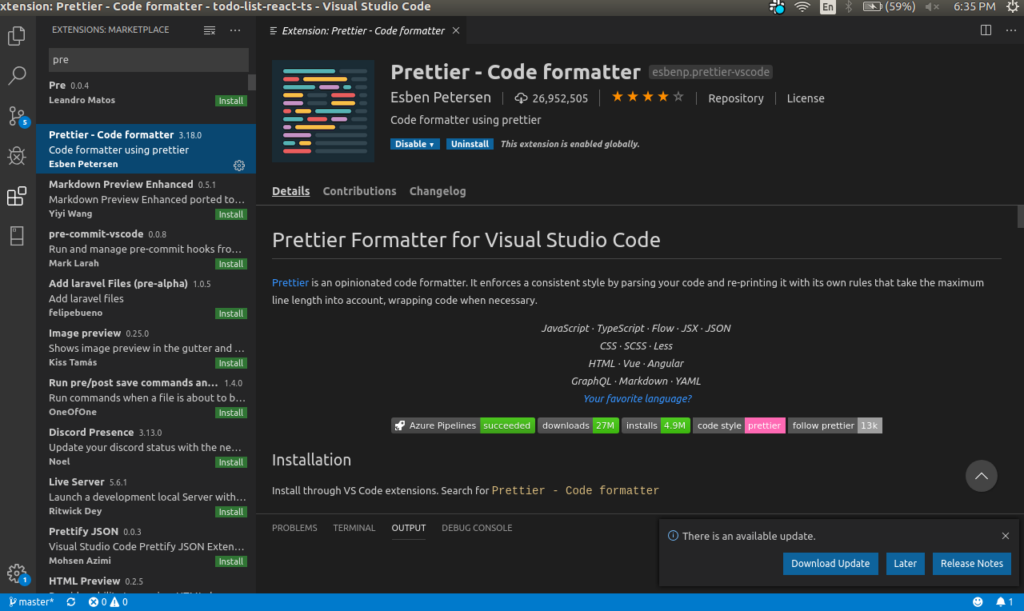
Now let’s install the necessary packages:
npm install --save-dev eslint-config-react-app @typescript-eslint/eslint-plugin@2.x @typescript-eslint/parser@2.x babel-eslint@10.x eslint@6.x eslint-plugin-flowtype@3.x eslint-plugin-<strong>import</strong>@2.x eslint-plugin-jsx-a11y@6.x eslint-plugin-react@7.x eslint-plugin-react-hooks@1.x
You can also use yarn.
Next, Create a .eslintrc.js file in your root directory. Below is a sample of what you can add in the .eslintrc.js file. You can change the rules and other settings as per your requirements.
module.exports = { parser: '@typescript-eslint/parser', // Specifies the ESLint parser extends: [ 'plugin:react/recommended', // Uses the recommended rules from @eslint-plugin-react 'plugin:@typescript-eslint/recommended', // Uses the recommended rules from @typescript-eslint/eslint-plugin ], parserOptions: { ecmaVersion: 2018, // Allows for the parsing of modern ECMAScript features sourceType: 'module', // Allows for the use of imports ecmaFeatures: { jsx: true, // Allows for the parsing of JSX }, }, rules: { // Place to specify ESLint rules. Can be used to overwrite rules specified from the extended configs // e.g. "@typescript-eslint/explicit-function-return-type": "off", }, settings: { react: { version: 'detect', // Tells eslint-plugin-react to automatically detect the version of React to use }, }, };
Now, to feature automatic fixing on Save.
Go to File → Preferences → Settings
And add in settings.json
“eslint.autoFixOnSave”: true
Below is a sample of how settings.json looks like:
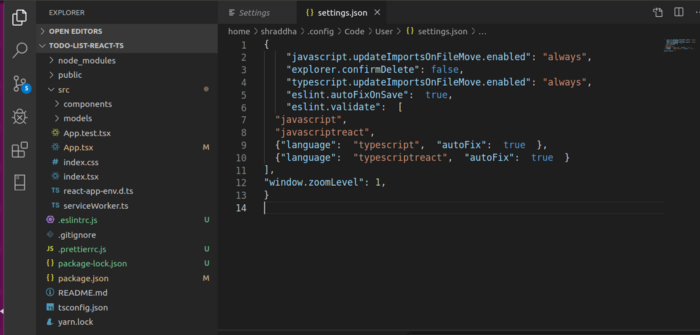
Now, let’s add prettier.
First, install the mandatory packages:
npm install prettier eslint-config-prettier eslint-plugin-prettier --dev
Create a .prettierrc.js file in the root directory of your project and add the following:
module.exports = { semi: true, trailingComma: 'all', singleQuote: true, printWidth: 120, tabWidth: 4, };
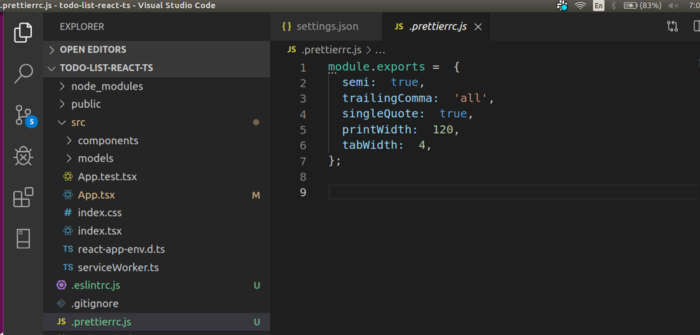
Lastly, Include prettier in the .eslintrc.js file:
In the extends section add:
‘plugin:prettier/recommended’
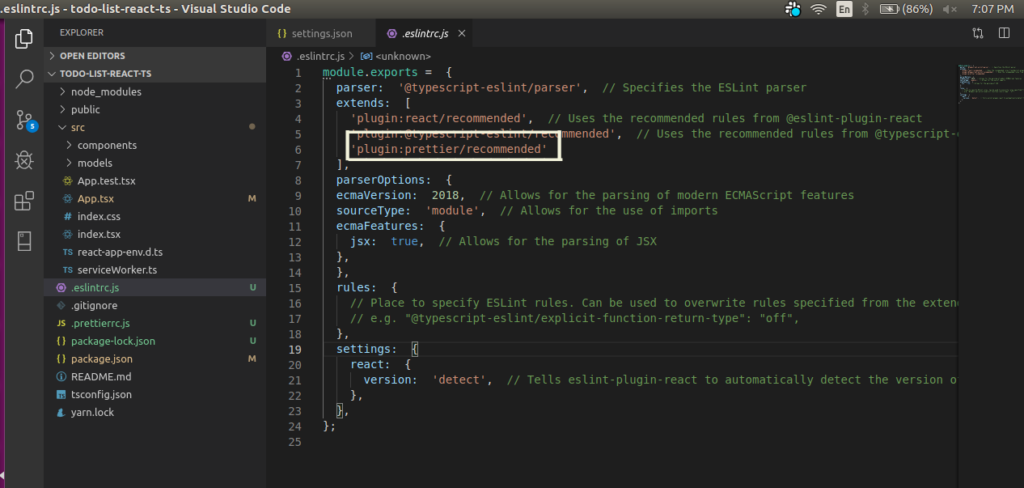
That’s it. You are good to go.
Team Udgama
- 10 backend mistakes (and their fixes) you must avoid as a Backend Developer
- 5 Frontend Trends You Can’t Ignore in 2022